【jQuery】Lightbox風のモーダルウィンドウを作成する
※本ページのリンクにはプロモーションが含まれています。
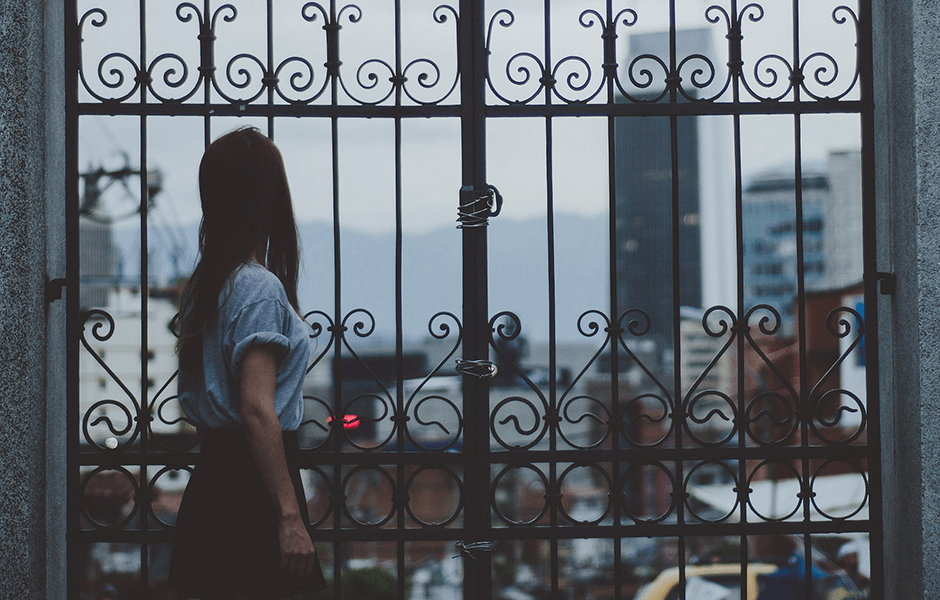
こんにちは、Ryohei(@ityryohei)です!
Lightboxが必要になった場合、ほとんどの案件でプラグインを使用しているのですが、画像やテキストを拡大するような単純なものであれば、プラグインに頼る必要もないように感じたため重い腰を上げて作成してみました。
今回はLightbox風のモーダルウィンドウの作成方法をご紹介したいと思います!
jQueryを読み込む
jQueryを利用するために、GoogleのCDNを利用してファイルを読み込みます。
CDNというのはContents Delivery Network(コンテンツ・デリバリー・ネットワーク)の略称で、ネットワークを経由してウェブコンテンツを利用するサービスを指します。ネットワークに接続されている機器であれば利用することができます。
CDNには有料、無料のものがありますが、jQueryのライブラリファイルは無料で利用できます。
jQueryのライブラリは以下の記述で読み込むことができます。
<script src="//ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script>
上記のスクリプトをheadタグ内に記述することで、jQueryを読み込むことができます。
モーダルウィンドウを作成する
今回作成するLightbox風のモーダルウィンドウは以下のデモでご確認いただけます。
HTML
HTMLではどの要素をクリックしたらモーダルウィンドウを表示するかと、モーダルウィンドウに表示する値等を決めておきます。
デモではcontentsItemというクラスを持っている要素がクリックされた際にモーダルウィンドウを表示するようにしています。
contentsItemはimgタグとpタグを持っています。これらの要素をモーダルウィンドウに表示します。
<main class="mainContents"> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample001.jpg" alt="sample"> <p>Item 001</p> </div> </main>
CSS
モーダルウィンドウのスタイルを定義します。
デモで使用しているlightBoxはコンテンツを表示する部分で、lightBoxBgがオーバーレイで表示する背景となっています。
両要素をfixedで固定してz-indexで重なり順を設定しています。
html, body { position: relative; width: 100%; height: 100%; margin: 0; padding: 0; background: #333; } img { width: 100%; } p { margin: 0; color: #fff; font-size: 20px; text-align: center; } /* lightBox表示時の背景 */ .lightBoxBg { position: fixed; top: 0; left: 0; display: none; min-width: 100%; min-height: 100%; overflow: hidden; background: rgba(0, 0, 0, 0.7); z-index: 10; } /* lightBox */ .lightBox { position: fixed; top: 50%; left: 50%; display: none; width: 95%; max-width: 1000px; height: auto; box-sizing: border-box; border: 5px solid #000; background: #000; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); z-index: 100; } .mainContents { width: 100%; height: auto; max-width: 1000px; margin: 0 auto; padding: 100px 0; clear: both; } .contentsItem { width: 96%; height: auto; margin: 0 2% 2% 2%; background: #23527c; cursor: pointer; } /* メディアクエリ */ @media screen and ( min-width: 768px ) { .contentsItem { width: 24%; height: 200px; margin: 0 1% 1% 0; background: #23527c; float: left; cursor: pointer; } .contentsItem:nth-child(4n) { margin: 0 0 1% 0; } }
JS
スクリプトは少し長くなってしまいましたので、コメントをご覧いただければと思います。
$(document).ready(function(){ //変数定義 var elem = '.contentsItem'; var wrap = 'body'; var lightBox = '.lightBox'; var lightBoxBg = '.lightBoxBg'; //elemがクリックされたら $(elem).on('click', function(){ //elemの子要素(img, p)から値を取得する var src = $(this).children('img').attr('src'); var text = $(this).children('p').text(); //wrapにlightBoxを追加する $(wrap).append('<div class="lightBoxBg"></div>'); $(wrap).append('<div class="lightBox"></div>'); //lightBoxに要素を追加する(他に追加する場合も同様) $(lightBox).append('<img src="" alt="sample">'); $(lightBox).append('<p></p>'); //elemの値を追加したlightBoxの要素に設定する $(lightBox).children('img').attr('src', src); $(lightBox).children('p').text(text); //lightBoxBgとlightBoxを表示する $(lightBoxBg).fadeIn('slow'); $(lightBox).fadeIn('slow'); //wrapのスクロールバーを非表示にする $(wrap).css('overflow', 'hidden'); }); //lightBox表示中にlightBoxBgがクリックされたら $(wrap).on('click', lightBoxBg , function(){ //lightBoxBgとlightBoxを削除する $(lightBoxBg).fadeOut('slow', function(){ $(this).remove(); }); $(lightBox).fadeOut('slow', function(){ $(this).remove(); }); //wrapのスクロールバーを表示する $(wrap).css('overflow', 'auto'); }); });
Copy & Paste
デモの全ソースになります!
<!doctype html> <html lang="ja"> <head> <meta charset="utf-8"> <title>Demo|Into the Program</title> <script src="//ajax.googleapis.com/ajax/libs/jquery/1.12.4/jquery.min.js"></script> <script> $(document).ready(function(){ var elem = '.contentsItem'; var wrap = 'body'; var lightBox = '.lightBox'; var lightBoxBg = '.lightBoxBg'; $(elem).on('click', function(){ var src = $(this).children('img').attr('src'); var text = $(this).children('p').text(); $(wrap).append('<div class="lightBoxBg"></div>'); $(wrap).append('<div class="lightBox"></div>'); $(lightBox).append('<img src="" alt="sample">'); $(lightBox).append('<p></p>'); $(lightBox).children('img').attr('src', src); $(lightBox).children('p').text(text); $(lightBoxBg).fadeIn('slow'); $(lightBox).fadeIn('slow'); $(wrap).css('overflow', 'hidden'); }); $(wrap).on('click', lightBoxBg , function(){ $(lightBoxBg).fadeOut('slow', function(){ $(this).remove(); }); $(lightBox).fadeOut('slow', function(){ $(this).remove(); }); $(wrap).css('overflow', 'auto'); }); }); </script> <style> html, body { position: relative; width: 100%; height: 100%; margin: 0; padding: 0; background: #333; } img { width: 100%; } p { margin: 0; color: #fff; font-size: 20px; text-align: center; } .lightBoxBg { position: fixed; top: 0; left: 0; display: none; min-width: 100%; min-height: 100%; overflow: hidden; background: rgba(0, 0, 0, 0.7); z-index: 10; } .lightBox { position: fixed; top: 50%; left: 50%; display: none; width: 95%; max-width: 1000px; height: auto; box-sizing: border-box; border: 5px solid #000; background: #000; -webkit-transform: translateX(-50%) translateY(-50%); transform: translateX(-50%) translateY(-50%); z-index: 100; } .mainContents { width: 100%; height: auto; max-width: 1000px; margin: 0 auto; padding: 100px 0; clear: both; } .contentsItem { width: 96%; height: auto; margin: 0 2% 2% 2%; background: #23527c; cursor: pointer; } @media screen and ( min-width: 768px ) { .contentsItem { width: 24%; height: 200px; margin: 0 1% 1% 0; background: #23527c; float: left; cursor: pointer; } .contentsItem:nth-child(4n) { margin: 0 0 1% 0; } } </style> </head> <body> <header> </header> <main class="mainContents"> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample001.jpg" alt="sample"> <p>Item 001</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample002.jpg" alt="sample"> <p>Item 002</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample003.jpg" alt="sample"> <p>Item 003</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample004.jpg" alt="sample"> <p>Item 004</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample005.jpg" alt="sample"> <p>Item 005</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample006.jpg" alt="sample"> <p>Item 006</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample007.jpg" alt="sample"> <p>Item 007</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample008.jpg" alt="sample"> <p>Item 008</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample009.jpg" alt="sample"> <p>Item 009</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample010.jpg" alt="sample"> <p>Item 010</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample011.jpg" alt="sample"> <p>Item 011</p> </div> <div class="contentsItem"> <img src="//into-the-program.com/demo/images/sample012.jpg" alt="sample"> <p>Item 012</p> </div> </main> </body> </html>
以上、Lightbox風のモーダルウィンドウを作成するのご紹介でした!
JavaScriptを基礎からしっかりと学びたい方へ
下記の参考書がおすすめです。私がJavaScript入門時に購入した書籍で、基礎から応用まで多様なサンプルを用いて解説されています。ページ数は多いですが、内容が伴っているのですらすら読めます。腰を据えて学びたい方に最良の一冊となっています。