【CSS/JS】スライドイン表示後にマスクがスライドアウトするアニメーション
※本ページのリンクにはプロモーションが含まれています。
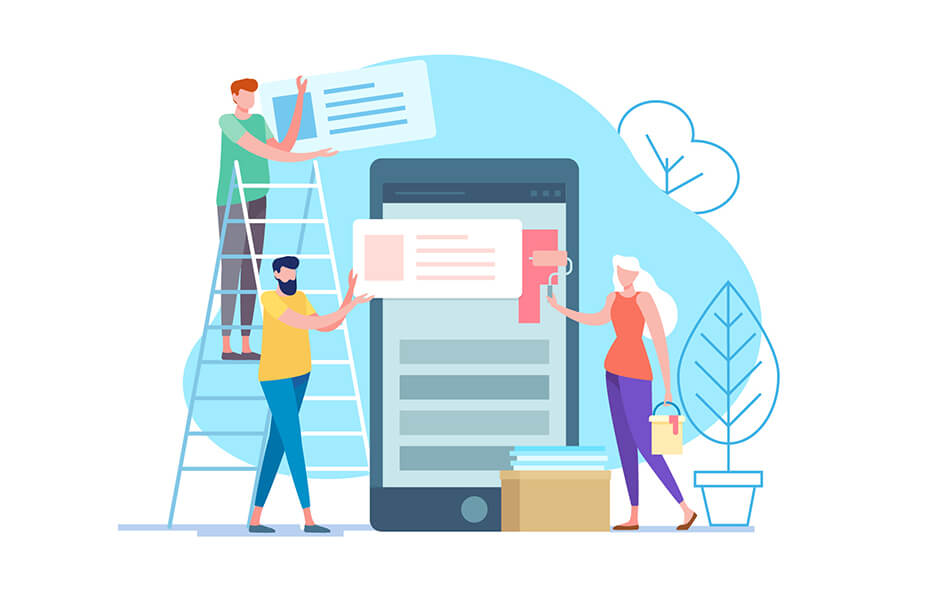
こんにちは、Ryohei(@ityryohei)です!
ボックスがスライドインで表示された後、重なり要素(マスク)がスライドアウトするアニメーションのご紹介です。
ページを下にスクロールしていくと、ボックスがスライドインで表示されます。その後、グラデーションの要素がスライドアウトしてコンテンツが表示されるアニメーションになります。
具体的には、下記のデモのような処理となります。
See the Pen Box Slide In Animation | jQuery by ryoy (@intotheprogram) on CodePen.
最近、このタイプのアニメーションが流行しているみたいで、似た表現を取り入れたサイトをよく見かけます。
今回、私なりに「こんな感じで実装しているのかな?」と考えながら、CSSとjQueryでスライドインアニメーションを実装してみました。同様の処理を検討している方の参考になれば幸いです。
本記事の内容
- HTMLとCSSでボックスを作成する
- CSSでアニメーションを設定する
- 疑似要素にマスクをつける
- jQueryでスクリプトを記述する
- ボックスをスライドインで表示してマスクを外すアニメーション
では解説していきます。
HTMLとCSSでボックスを作成する
スライドインで示する要素を作成していきます。
HTML
まずはHTMLでベースになるタグを記述します。本記事では、「box」という要素に「boxInner」という要素を持たせています。
<div class="box"> <div class="boxInner">Lorem Ipsum</div> </div>
CSS
続いて、CSSでスタイルを定義していきます。CSSで重要なのは下記の点です。
- 実際にスライドインで表示されるのは「box」の子要素である「boxInner」のため、「boxInner」が「box」外で表示されないようにoverflowを非表示にしておく
- 「box」には幅や高さや余白を指定、「boxInner」には背景等は指定する
- ページ読み込み時に「boxInner」は非表示にするため「opacity: 0」を設定する
上記を考慮して、CSSを記述します。
.box { width: 100%; height: 200px; margin: 0 0 2% 0; overflow: hidden; } .box .boxInner { width: inherit; height: inherit; line-height: 200px; font-size: 1.5em; text-align: center; color: #555; background-color: #fff; opacity: 0; }
CSSでアニメーションを設定する
続いて、CSSのkeyframesでアニメーションを設定します。設定するアニメーションは下記の2種類になります。
- 「boxInner」がスライドイン表示されるアニメーション
- マスク要素がスライドアウトするアニメーション
マスク要素というのは、デモでいうところのグラデーションが設定された重なり要素になります。「boxInner」がスライドインした後、マスクがスライドアウトするようにアニメーションを設定します。
//「boxInner」をスライドインする @keyframes play { from { transform: translateX(-100%); } to { transform: translateX(0); } } //マスク要素をスライドアウトする @keyframes maskOut { from { transform: translateX(0); } to { transform: translateX(100%); } }
アニメーションを実行するclassを定義する
CSSの設定はこちらで最後になります。
アニメーションを実行するための「isPlay」というclassを定義します。
「isPlay」にスライドインを実行するためのanimationプロパティを指定します。併せて、「isPlay」の疑似要素にグラデーションのマスクを付加します。
「isPlay」の疑似要素にマスクを付加することで、スライドイン表示後、一定時間コンテンツを隠すことができ、スライドアウトのアニメーションも簡単に設定することができます。
//スライドインを動作するclass .isPlay { animation-name: play; animation-duration: .5s; animation-fill-mode: forwards; animation-timing-function: cubic-bezier(.8,0,.5,1); position: relative; opacity: 1 !important; } //isPlayの疑似要素にマスクとマスクを外すアニメーションを設定 .isPlay:before { animation-name: maskOut; animation-duration: .5s; animation-delay: .5s; animation-fill-mode: forwards; animation-timing-function: cubic-bezier(.8,0,.5,1); content: ''; position: absolute; top: 0; left: 0; z-index: 1; width: 100%; height: 100%; background-image: linear-gradient( 109.6deg, rgba(156,252,248,1) 11.2%, rgba(110,123,251,1) 91.1% ); }
jQueryでスクリプトを記述する
最後に、jQueryでスクリプトを追加します。
内容としては、スクロール中に表示された「boxInner」に「isPlay」を付与してスライドイン表示するというものになります。
$(window).on('load scroll', function(){ var elem = $('.boxInner'); elem.each(function () { var isPlay = 'isPlay'; var elemOffset = $(this).offset().top; var scrollPos = $(window).scrollTop(); var wh = $(window).height(); if(scrollPos > elemOffset - wh + (wh / 4)){ $(this).addClass(isPlay); } }); });
スライドイン表示後にマスクがスライドアウトするアニメーション
下記が完成版のデモになります。
See the Pen Box Slide In Animation | jQuery by ryoy (@intotheprogram) on CodePen.
まとめ
最後に、デモで使用しているソースをまとめます。下記を利用することでボックススライドインのアニメーションを実装することができます。
<!-- CSS --> <style> body { background-color: #2f3640; } .container { display: flex; flex-wrap: wrap; justify-content: space-between; width: 100%; max-width: 700px; height: auto; margin: 0 auto; padding: 40px 0; } .box { width: 100%; height: 200px; margin: 0 0 2% 0; overflow: hidden; } .box .boxInner { width: inherit; height: inherit; line-height: 200px; font-size: 1.5em; text-align: center; color: #555; background-color: #fff; opacity: 0; } .isPlay { animation-name: play; animation-duration: .5s; animation-fill-mode: forwards; animation-timing-function: cubic-bezier(.8,0,.5,1); position: relative; opacity: 1 !important; } .isPlay:before { animation-name: maskOut; animation-duration: .5s; animation-delay: .5s; animation-fill-mode: forwards; animation-timing-function: cubic-bezier(.8,0,.5,1); content: ''; position: absolute; top: 0; left: 0; z-index: 1; width: 100%; height: 100%; background-image: linear-gradient( 109.6deg, rgba(156,252,248,1) 11.2%, rgba(110,123,251,1) 91.1% ); } @keyframes play { from { transform: translateX(-100%); } to { transform: translateX(0); } } @keyframes maskOut { from { transform: translateX(0); } to { transform: translateX(100%); } } </style> <!-- HTML --> <section class="container"> <div class="box"> <div class="boxInner">Lorem Ipsum</div> </div> </section> <!-- Script --> <script src="https://code.jquery.com/jquery-3.3.1.min.js"></script> <script> $(window).on('load scroll', function(){ var elem = $('.boxInner'); elem.each(function () { var isPlay = 'isPlay'; var elemOffset = $(this).offset().top; var scrollPos = $(window).scrollTop(); var wh = $(window).height(); if(scrollPos > elemOffset - wh + (wh / 4)){ $(this).addClass(isPlay); } }); }); </script>
本記事でご紹介した内容とは異なりますが、下記で似たようなアニメーションをご紹介していますので、お時間があればご覧ください。
【CSS/JS】画像上の要素がカーテンのように開くスクロールアニメーション
以上、スライドイン表示後にマスクがスライドアウトするアニメーションのご紹介でした!
ボックススライドインのアニメーションはワンポイントとしても全体に適用しても違和感のないアニメーションですので、色々なサイトでご活用いただければ幸いです。